netneurotools.stats.permtest_1samp
- netneurotools.stats.permtest_1samp(a, popmean, axis=0, n_perm=1000, seed=0)[source]
Non-parametric equivalent of
scipy.stats.ttest_1samp()
.Generates two-tailed p-value for hypothesis of whether a differs from popmean using permutation tests
- Parameters:
a (array_like) – Sample observations
popmean (float or array_like) – Expected valued in null hypothesis. If array_like then it must have the same shape as a excluding the axis dimension
axis (int or None, optional) – Axis along which to compute test. If None, compute over the whole array of a. Default: 0
n_perm (int, optional) – Number of permutations to assess. Unless a is very small along axis this will approximate a randomization test via Monte Carlo simulations. Default: 1000
seed ({int, np.random.RandomState instance, None}, optional) – Seed for random number generation. Set to None for “randomness”. Default: 0
- Returns:
stat (float or numpy.ndarray) – Difference from popmean
pvalue (float or numpy.ndarray) – Non-parametric p-value
Notes
Providing multiple values to popmean to run independent tests in parallel is not currently supported.
The lowest p-value that can be returned by this function is equal to 1 / (n_perm + 1).
Examples
>>> from netneurotools import stats >>> np.random.seed(7654567) # set random seed for reproducible results >>> rvs = np.random.normal(loc=5, scale=10, size=(50, 2))
Test if mean of random sample is equal to true mean, and different mean. We reject the null hypothesis in the second case and don’t reject it in the first case.
>>> stats.permtest_1samp(rvs, 5.0) (array([-0.985602 , -0.05204969]), array([0.48551449, 0.95904096])) >>> stats.permtest_1samp(rvs, 0.0) (array([4.014398 , 4.94795031]), array([0.00699301, 0.000999 ]))
Example using axis and non-scalar dimension for population mean
>>> stats.permtest_1samp(rvs, [5.0, 0.0]) (array([-0.985602 , 4.94795031]), array([0.48551449, 0.000999 ])) >>> stats.permtest_1samp(rvs.T, [5.0, 0.0], axis=1) (array([-0.985602 , 4.94795031]), array([0.51548452, 0.000999 ]))
Examples using netneurotools.stats.permtest_1samp
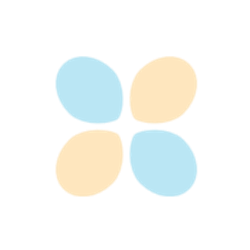
Non-parametric significance testing with permutations